Breakthrough alert! “Maximize Speed: The Heap Sort Advantage in 2023” unlocks the formula for algorithmic speed, setting the pace for a new era.
Table of Contents
- Introduction
- Heap Sort Fundamentals
- The Need for Speed: Heap Sort in Action
- Key Advantages of Heap Sort in 2023
- Code
- Conclusion
Introduction
In the ever-evolving landscape of algorithmic efficiency, one stalwart stands out: Heap Sort. This article delves into the intricacies of Heap Sort, exploring its fundamentals, real-world applications, and key advantages, with a keen eye on the technological horizon of 2023.
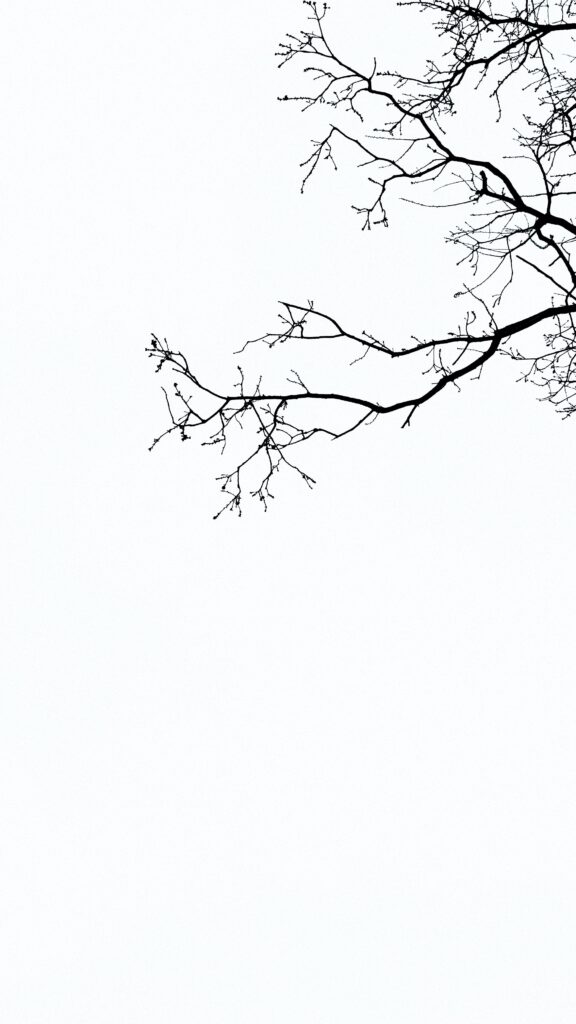
Heap Sort Fundamentals
Understanding Heap Data Structures
Heap Sort’s prowess lies in its adept utilization of heap data structures. These structures, binary trees with special ordering properties, come in two distinct flavors: Min Heap and Max Heap. The former ensures that the parent node is smaller than its children, while the latter enforces the opposite. This inherent distinction forms the backbone of Heap Sort’s efficiency.
Visualization of Heap Structures
To truly grasp the power of Heap Sort, visualizing heap structures becomes paramount. Picture a hierarchical arrangement where every node follows the heap property, creating a systematic order that facilitates efficient sorting.
Exploring the Heap Sort Algorithm
At the heart of Heap Sort lies a robust algorithm, elegantly designed for optimal performance. Heapify, the core mechanism, organizes data into a heap structure, setting the stage for subsequent sorting maneuvers. Sifting up and down strategies further refine the arrangement, ensuring the sorted output we seek.
The Need for Speed: Heap Sort in Action
Real-world Application of Heap Sort
Heap Sort transcends theoretical elegance, finding practical applications in the sorting of massive datasets. Its efficiency becomes pronounced when dealing with extensive and complex information, making it a go-to solution in various industries.
Sorting Massive Datasets
The ability to swiftly and accurately sort massive datasets is where Heap Sort shines. Its time complexity, coupled with the unique structure of heap data, allows for unparalleled speed and reliability in handling voluminous information sets.
Optimizing Resource-Intensive Processes
In resource-intensive processes, where efficiency is the name of the game, Heap Sort emerges as a key player. Its strategic algorithms cut through the computational clutter, optimizing resource utilization and streamlining operations.
Case Studies: Successful Implementations in 2023
Delving into the current landscape, we examine industry examples showcasing the tangible speed gains facilitated by Heap Sort. Performance metrics and comparisons paint a vivid picture of its real-world impact, solidifying its relevance in 2023.
Key Advantages of Heap Sort in 2023
Scalability and Big-O Notation Breakdown
Heap Sort’s advantages extend beyond the surface, unraveling in its scalability and Big-O notation breakdown. An in-depth analysis of time and space complexity unveils its efficiency in varying scenarios, establishing it as a versatile solution.
Along with Merge Sort and Quick Sort, Heap Sort also has the time complexity of O(nlogn)
Analyzing Time Complexity
In the race against time, Heap Sort emerges as a frontrunner. Analyzing its time complexity provides insights into its rapid sorting capabilities, making it a strategic choice in time-sensitive applications.
Evaluating Space Complexity
Heap Sort’s prowess isn’t limited to time efficiency alone. Evaluating its space complexity reveals a balanced algorithm, efficiently utilizing memory resources without unnecessary overhead.
Adaptive Versatility
One of Heap Sort’s standout features is its adaptive versatility. Handling variable input sizes with finesse, it remains effective whether sorting a handful of elements or grappling with extensive datasets.
Implications for Dynamic Programming
Heap Sort’s adaptability extends to dynamic programming environments. Its implications in dynamic scenarios underscore its relevance in modern, ever-changing computational landscapes.
Code
Max Heap
def heapify(unsorted, index, heap_size):
largest = index
left_index = 2 * index + 1
right_index = 2 * index + 2
if left_index < heap_size and unsorted[left_index] > unsorted[largest]:
largest = left_index
if right_index < heap_size and unsorted[right_index] > unsorted[largest]:
largest = right_index
if largest != index:
unsorted[largest], unsorted[index] = unsorted[index], unsorted[largest]
heapify(unsorted, largest, heap_size)
def heap_sort(unsorted):
n = len(unsorted)
for i in range(n // 2 - 1, -1, -1):
heapify(unsorted, i, n)
for i in range(n - 1, 0, -1):
unsorted[0], unsorted[i] = unsorted[i], unsorted[0]
heapify(unsorted, 0, i)
return unsorted
if __name__ == "__main__":
user_input = input("Enter numbers separated by a comma:\n").strip()
unsorted = [int(item) for item in user_input.split(",")]
print(heap_sort(unsorted))
Min Heap
def heapify(unsorted, index, heap_size):
largest = index
left_index = 2 * index + 1
right_index = 2 * index + 2
if left_index < heap_size and unsorted[left_index] < unsorted[largest]:
largest = left_index
if right_index < heap_size and unsorted[right_index] < unsorted[largest]:
largest = right_index
if largest != index:
unsorted[largest], unsorted[index] = unsorted[index], unsorted[largest]
heapify(unsorted, largest, heap_size)
def heap_sort(unsorted):
n = len(unsorted)
for i in range(n // 2 - 1, -1, -1):
heapify(unsorted, i, n)
for i in range(n - 1, 0, -1):
unsorted[0], unsorted[i] = unsorted[i], unsorted[0]
heapify(unsorted, 0, i)
return unsorted
if __name__ == "__main__":
user_input = input("Enter numbers separated by a comma:\n").strip()
unsorted = [int(item) for item in user_input.split(",")]
print(heap_sort(unsorted))
Visit for more DSA -> Github
Conclusion
Recap of Heap Sort’s Timeless Relevance
In closing, we revisit Heap Sort’s timeless relevance. Its robust fundamentals, real-world applications, and adaptive advantages underscore its enduring significance in the world of algorithmic efficiency.
Emphasizing its Pivotal Role in Accelerating Algorithms in 2023
As we step into 2023, Heap Sort’s pivotal role in accelerating algorithms becomes more pronounced than ever. A testament to its adaptability and efficiency, Heap Sort stands as a beacon in the ever-advancing realm of computational prowess.