Uncover the power of idempotency in API design. Eliminate duplicates, ensure reliability, and achieve seamless operations. Dive into the world of zero duplicates and all results
Table of Contents
- Introduction
- Unveiling Idempotency
- Idempotency in Action
- Benefits of Idempotency
- Implementing Idempotency
- Idempotency and Data Integrity
- Common Misconceptions
- Best Practices
- Real-world Examples
- Future of Idempotency in APIs
- Conclusion
Introduction
In the unexpectedly expanding region of software program development, wherein velocity, performance, and dependability are crucial, the understated however important idea of smooth API operations takes center stage. The riding cause at the back of accomplishing this harmony is idempotency, a time period that can sound technical but is essential to getting rid of duplicates and making sure of unwavering balance in the API environment.
Unveiling Idempotency
Defining Idempotency: A Clear Breakdown
Idempotency is basically the idea that regardless of how usually a motion is performed, the outcome always stays equal. In other phrases, whether or not a nicely-based idempotent operation is achieved once or 100 times, the end result will always be the same. This innate characteristic makes APIs considerably extra reliable and predictable.
Why Idempotency Matters in API Design
Do not forget software that approaches orders for net purchasing. Without idempotence, a duplicate order request may want to bring about an accidental double price for a customer, shattering consideration and alienating customers. Idempotence protects against such mistakes by making certain that each motion will result in the preferred result regardless of the condition.
Real-World Scenarios: Challenges Without Idempotency
Think about a banking software where money is moved between money owed. Without an idempotent system, users may retry a transaction if a network blunder prevents it from being shown, resulting in numerous transfers as soon as connectivity is restored. In the absence of idempotence, the surroundings are chaotic, making it difficult to get to the bottom of incorrect transactions.
Idempotency in Action
How Idempotency Works: Step-by-Step Process
To recognize how idempotency capabilities, do not forget an API request to adjust a consumer’s e-mail address. No matter how frequently this request is made, the e-mail cope is in the end most effectively changed as soon as. This simple but vital characteristic ensures that retrying procedures won’t have any poor repercussions and fosters sincere surroundings.
Understanding Idempotent Requests and Their Impact
Idempotent requests have an effect that extends beyond information consistency. So as to permit idempotent behaviors throughout numerous utility layers, they’re vital. This idea covers areas like caching, synchronization, and database integrity in which repeated activities ought to cause confusion.
Benefits of Idempotency
Zero Duplicates, Maximum Results: The Main Advantage
The primary benefit of idempotency is pleasantly summed up by means of the term “zero duplicates”. Idempotency guarantees that several same requests result in the identical reaction as an unmarried request, hence putting off the hazard of unintended redundancies. This guarantees that moves have the intended impact while not having unintended side consequences.
Consistency in Data: Building Trust Among Users
In a global where information rules, consistency of information is vital. Idempotence guarantees that a consumer’s request might be answered in a manner this is steady with its supposed utilization. This promotes consideration with the aid of raising users’ perspectives of reliability and professionalism.
Error Management and Prevention: Reducing Complexity
Idempotency is crucial for coping with both unsuccessful and hit operations. While an idempotent request fails, builders can confidently retry without demanding unanticipated consequences. Via notably decreasing the complexity of error-handling processes, this system makes maintenance and troubleshooting a good deal simpler.
Implementing Idempotency
Design Principles for Idempotent APIs
Idempotent API improvement calls for both foresight and accuracy. Builders should focus on developing approaches that produce predictable effects while repeated. Following the idempotency guidelines makes making sure that moves like updating person profiles or making reservations produce steady outcomes.
Idempotency Keys: Ensuring Uniqueness in Requests
Idempotency often depends on one-of-a-kind identities, or “idempotency keys,” related to requests. Those keys act as gatekeepers, ensuring that an operation is diagnosed because they are equal even if it’s miles submitted more than once. The dependability of idempotent actions is ensured by way of deciding on sturdy idempotency keys and efficaciously maintaining them.
Setup Flask and Redis
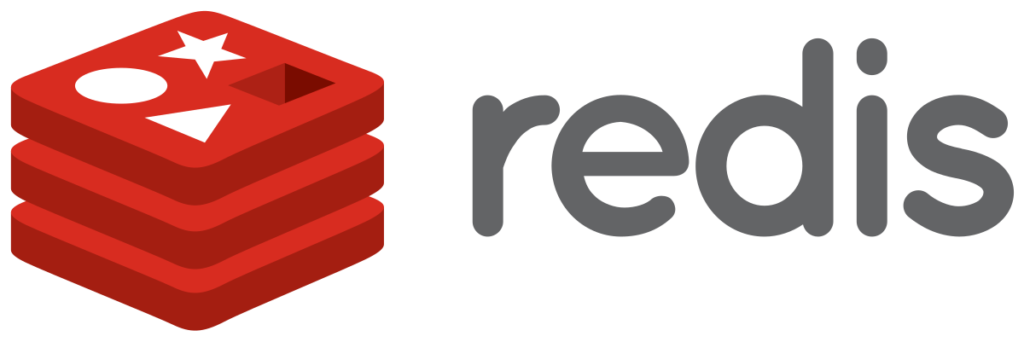
- Set Up Flask and Redis: Start setting up Flask application with Redis. Redis is the most used database for Idempotency, because of its in-memory storage. You can import redis or Flask-Redis.
- Check Idempotency: Check for Idempotency keys in Redis before processing the request. If exists, the request is already processed and get the processed result.
- Process Request: If the idempotency key doesn’t exist in Redis, process the request and get the result.
- Store Idempotency Key: After successfully processing the request, store the idempotency key in Redis along with the response data. Use an appropriate expiration time based on your application’s needs.
Install Redis on Windows
Redis is not officially supported on Windows. However, you can install Redis on Windows using WSL2 (Windows Subsystem for Linux)
Install or enable WSL2
Microsoft provides detailed instructions for installing WSL. Follow these instructions, and take note of the default Linux distribution it installs. This guide assumes Ubuntu.
Install Redis
curl -fsSL https://packages.redis.io/gpg | sudo gpg --dearmor -o /usr/share/keyrings/redis-archive-keyring.gpg
echo "deb [signed-by=/usr/share/keyrings/redis-archive-keyring.gpg] https://packages.redis.io/deb $(lsb_release -cs) main" | sudo tee /etc/apt/sources.list.d/redis.list
sudo apt-get update
sudo apt-get install redis
Start Redis
sudo service redis-server start
Connect Redis for Testing
redis-cli
127.0.0.1:6379> ping
PONG
Code Implementation in Flask(post request)
from flask import Flask, request, jsonify
from flask_redis import FlaskRedis
app = Flask(__name__)
app.config['REDIS_URL'] = "redis://localhost:6379/0"
redis = FlaskRedis(app)
@app.route('/idempotent-resource', methods=['POST'])
def idempotent_resource():
idempotency_key = request.headers.get('Idempotency-Key')
if idempotency_key:
response = redis.get(idempotency_key)
if response:
return jsonify(response), 200
# Process the actual request
data = request.get_json()
# Perform operations, update database, generate response
response = {"message": "Resource created successfully"}
# Store response with idempotency key in Redis
if idempotency_key:
redis.setex(idempotency_key, 3600, response)
return jsonify(response), 201
if __name__ == '__main__':
app.run()
Code Implementation in Flask(get request)
redis_DB.py
import redis
import json
from datetime import datetime,timedelta
class Redis_DB(object):
def __init__(self):
#Input - User Id
#Output - Result/False
#Establish Connection
redis_host = "localhost"
redis_port = 6379
redis_password = ""
self.engine = redis.Redis(host=redis_host, port=redis_port, password=redis_password)
self.engine.flushall()
def main(self,uid):
#red.insert(1,'Hello BK')
msg = self.retrieve(uid)
if msg:
msg_str = msg.decode("utf-8")
msg_dict = json.loads(msg_str)
result = self.verify(msg_dict,1)
if result:
return msg_dict['Result']
else:
return False
else:
return False
def insert(self,uid, result):
#Inser new records into Redis
current_time = datetime.now().strftime("%m/%d/%Y %H:%M:%S")
value = {}
value['Time'] = current_time
value['Result'] = result
self.engine.set(uid, json.dumps(value))
def retrieve(self,uid):
msg = self.engine.get(uid)
if msg:
return msg
else:
return None
def verify(self,value,uid):
#Verify the Last Processed Time. If more than 2 mins reprocess, else use same result
db_time = datetime.strptime(value['Time'],"%m/%d/%Y %H:%M:%S")
difference_time = (datetime.now() - db_time).total_seconds()
if difference_time > 120:
self.engine.delete(uid)
return False
else:
return True
controller.py
import json
from controller.redis_DB import *
from flask import Flask, request
app = Flask(__name__)
red = Redis_DB()
@app.route('/')
def home():
return 'Welcome to Idempotency'
@app.route('/movies/', methods=['GET'])
def request_movies():
uid = request.args.get('uid')
response = red.main(uid)
if not response:
response = []
with open('database/movies.json','r') as f:
movie = json.loads(f.read())
for i in movie:
response.append(i['Movie'])
red.insert(uid,response)
else:
return json.dumps(response)
return json.dumps(response)
To get the complete code: Chekout the My Github – Idempotency
Idempotency and Data Integrity
Maintaining Data Integrity: The Role of Idempotency
Preserving the integrity of information is vital in information-driven surroundings. Idempotency, which guarantees that repeated acts do not bring about undesirable modifications, is important in this situation. Idempotency protects data accuracy whether or not it’s converting a user’s personal information or changing inventory counts.
Ensuring Accurate Updates: Idempotency’s Impact on Resource Modification
Think about an API request that modifies the product amount in an internet keeper’s inventory. Because of idempotency, despite the fact that the request is issued again because of community trouble, the final result is identical: just one alternate is made to the amount. By means of ensuring specific aid changes, the kingdom of the utility is kept consistent.
Common Misconceptions
Myth-Busting: Addressing Misconceptions About Idempotency
Even though it is a strong concept, idempotence is regularly cloaked in rumors. One such false impression is the idea that caching and idempotency are the same aspect. To fully make use of idempotency in API architecture, it is critical to address these misunderstandings.
Best Practices
Guidelines for Developers: Writing Idempotent Code
Adherence to great practices is needed which will create idempotent APIs. Developers ought to carefully craft processes that now not simplest deliver the preferred outcomes but additionally hold consistency while repeated. This necessitates careful attention to information validation, mistakes management, and state modifications.
Versioning and Backward Compatibility: Long-Term Considerations
The difficulty of preserving idempotency between versions emerges as APIs develop. Cautious versioning techniques are wished so as to strike a stability between innovation and stability. Backward compatibility ensures that idempotent behaviors are preserved even when new capabilities are brought.
Real-world Examples
Success Stories: Companies Benefiting from Idempotent APIs
A few enterprise titans used idempotent APIs to enhance their services. Those achievement stories exhibit how idempotency contributes to dependable, consumer-pleasant reviews, from payment structures assuring faultless transactions to social media platforms stopping reproduction posts.
Case Study: Implementing Idempotency to Enhance User Experience
Explore a case examination of a startup that advanced its personal experience which include idempotency in the architecture of its API. Have a look at the problems they encountered, the techniques they used to guarantee consistency, and the first-rate improvement in client happiness that followed.
Future of Idempotency in APIs
Evolving Trends: Idempotency’s Role in Modern API Architecture
Idempotency turns into greater importance as the era advances. Idempotent behaviors are getting more and more vital to the stableness, scalability, and predictability of current API architectures. Discover the new trends exhibiting how idempotency is vital for influencing the virtual environment.
Predictions for the API Landscape: Scaling with Idempotency
Looking ahead, it’s miles viable to peer that idempotency becomes a key component of API scaling techniques. Processing API calls without developing mistakes turns increasingly crucial as their volume soars. Idempotence is about to play a key position in navigating this unstable panorama.
Conclusion
Recap: Idempotency’s significance in API development
Idempotency shines as a ray of actuality within the global of APIs, where consistency and dependability are essential. It’s far an important tool for builders running to build resilient structures because of its capability to take away duplicates, keep records integrity, and make blunders management simple.
Empowering Reliability: Encouraging Adoption of Idempotent Practices
Because the curtain falls in this exploration of idempotency in API design, the message is apparent: adopting idempotent practices is not just a preference; it’s a need. In a landscape in which seamless operations and unwavering reliability are non-negotiable, embracing idempotency empowers developers to pave the way for a future built on consistency and trust.